In our previous experiment, we explored join operations and table partitioning to manage duplicate bookings in our flight booking system. While these methods were effective, they came with certain complexities and costs. To further optimize our system, we turned to hash-based techniques. This article will walk you through the development and implementation of MD5-based hashing for efficient duplicate checking.
Initially, join operations and table partitioning provided a foundation for handling duplicate data. However, their resource-intensive nature and the associated costs prompted us to seek more efficient solutions. Hash-based techniques, specifically MD5-based hashing, emerged as a promising alternative.
The MD5 hashing algorithm offers a way to transform passenger data into unique hash values. By generating these hash values, we could quickly compare and identify duplicate entries without the need for complex joins. This approach significantly reduced the processing time and improved the system’s overall performance.
The development process began with identifying the key fields that contribute to duplicate records. These fields were combined to create a composite string, which was then hashed using the MD5 algorithm. Storing these hash values alongside the original records allowed for rapid duplicate checks by simply comparing the hashes.
Implementing MD5-based hashing required modifications to our database schema and the introduction of new procedures for data insertion and updating. Each new record underwent hashing before being inserted, ensuring that duplicates were identified and addressed in real time.
Benchmarking this new approach against our previous methods showed substantial improvements in both speed and accuracy. The hash-based technique outperformed traditional joins and partitioning, making it a viable solution for our growing data needs.
As we continue to refine our system, future articles will delve deeper into the specific steps and code implementations used in this MD5-based hashing technique. These insights will help others optimize their data management processes for better performance and efficiency. Stay tuned for these detailed guides to enhance your understanding of advanced duplicate detection methods.
Hash-Based Techniques
The idea behind using hash-based techniques for duplicate checking is to create a unique hash value for each record’s relevant data fields. By comparing these hash values instead of the raw data, we can quickly identify duplicates with minimal computational overhead.
Key Advantages:
- Speed: Hash comparisons are faster than comparing multiple fields individually.
- Efficiency: Reduced computational load as hash values are shorter and easier to compare.
- Simplicity: Hashing algorithms like MD5 are straightforward to implement and integrate.
Selecting the Right Hashing Algorithm
We chose MD5 for its balance of speed and simplicity. Although MD5 is not suitable for cryptographic security, it is adequate for our purpose of identifying duplicate records.
Hashing the Data
Next, we decided which fields to include in the hash. For our flight booking system, the key fields were:
firstName, lastName, dob, passportNumber, flightNumber, departureDateTime
By combining these fields, we created a single string for each record to hash.
Code to Generate MD5 Hash:
import hashlib
def generateMd5Hash(firstName, lastName, dob, passportNumber, flightNumber, departureDateTime):
hashInput = f"{firstName}{lastName}{dob}{passportNumber}{flightNumber}{departureDateTime}"
return hashlib.md5(hashInput.encode()).hexdigest()
Storing Hash Values in the Database
We added a new column booking_hash
to our Passengers
table to store the MD5 hash of each record. Additionally, we set this column to be unique to prevent duplicate entries.
SQL Command to Add Column:
ALTER TABLE Passengers ADD COLUMN booking_hash VARCHAR(32) UNIQUE;
Implementing Duplicate Check on Insert
Before inserting a new record, we generate the booking_hash
and check if it already exists in the Passengers
table. If it exists, the insertion is rejected.
Code for Insertion:
def insertNewPassenger(firstName, lastName, dob, passportNumber, flightNumber, departureDateTime):
bookingHash = generateMd5Hash(firstName, lastName, dob, passportNumber, flightNumber, departureDateTime)
conn = psycopg2.connect("dbname=flightdb user=postgres password=secret")
cur = conn.cursor()
cur.execute("SELECT COUNT(*) FROM Passengers WHERE booking_hash = %s", (bookingHash))
if cur.fetchone()[0] > 0:
print("Duplicate booking detected. Insertion rejected.")
else:
cur.execute(
"""
INSERT INTO Passengers (firstName, lastName, dob, passportNumber, flightNumber, departureDateTime, booking_hash)
VALUES (%s, %s, %s, %s, %s, %s, %s)
""",
(firstName, lastName, dob, passportNumber, flightNumber, departureDateTime, bookingHash)
)
conn.commit()
cur.close()
conn.close()
Performance Testing and Optimization
We conducted performance tests to compare the new hash-based method with our previous approaches. The results showed a significant reduction in query execution time and resource usage.
Performance Metrics:
- Query Runtime: Reduced to under 0.5 seconds for 1 million records.
- CPU Usage: Reduced to 5% during duplicate checks.
- Memory Usage: Reduced to 100 MB of additional RAM.
- Server Cost: With optimized performance, server requirements decreased, resulting in much lower operational costs.
While this approach does increase computation slightly due to the hash generation process, the overall efficiency gains make it a worthwhile trade-off. Additionally, one potential drawback is that the data used to generate the hash must be accurate and consistent with previous bookings to ensure reliable duplicate detection.
Evaluation
Benefits
- Speed and Efficiency: Hash comparisons are much faster than comparing multiple fields individually. This significantly reduces query execution time, making the system more responsive.
- Reduced Computational Load: Hash values are shorter and easier to compare than raw data, leading to lower CPU and memory usage.
- Simplicity: Implementing MD5 hashing is straightforward and can be easily integrated into existing systems.
- Cost-Effective: By optimizing performance, the system’s overall server requirements decrease, leading to lower operational costs.
- Scalability: This method can handle large datasets efficiently, making it suitable for high-traffic systems.
Potential Drawbacks
- Increased Computation: While the overall efficiency gains are significant, there is a slight increase in computation due to the hash generation process.
- Data Accuracy Requirement: The data used to generate the hash must be accurate and consistent. Any discrepancies in the data fields (e.g., minor typos) could lead to different hash values, potentially allowing duplicates to slip through.
- Non-Cryptographic: MD5 is not suitable for cryptographic purposes, but for duplicate detection, its speed and simplicity make it a good fit despite its vulnerabilities.
Implementing MD5-based hashing for duplicate checking proved to be a highly efficient and cost-effective solution. This method not only simplified the process but also significantly improved the system’s performance. By leveraging hash-based techniques, we achieved a more streamlined and reliable flight booking system.
This concludes my series on managing duplicate bookings. Keep in touch for any future articles and more insights into optimizing database systems!
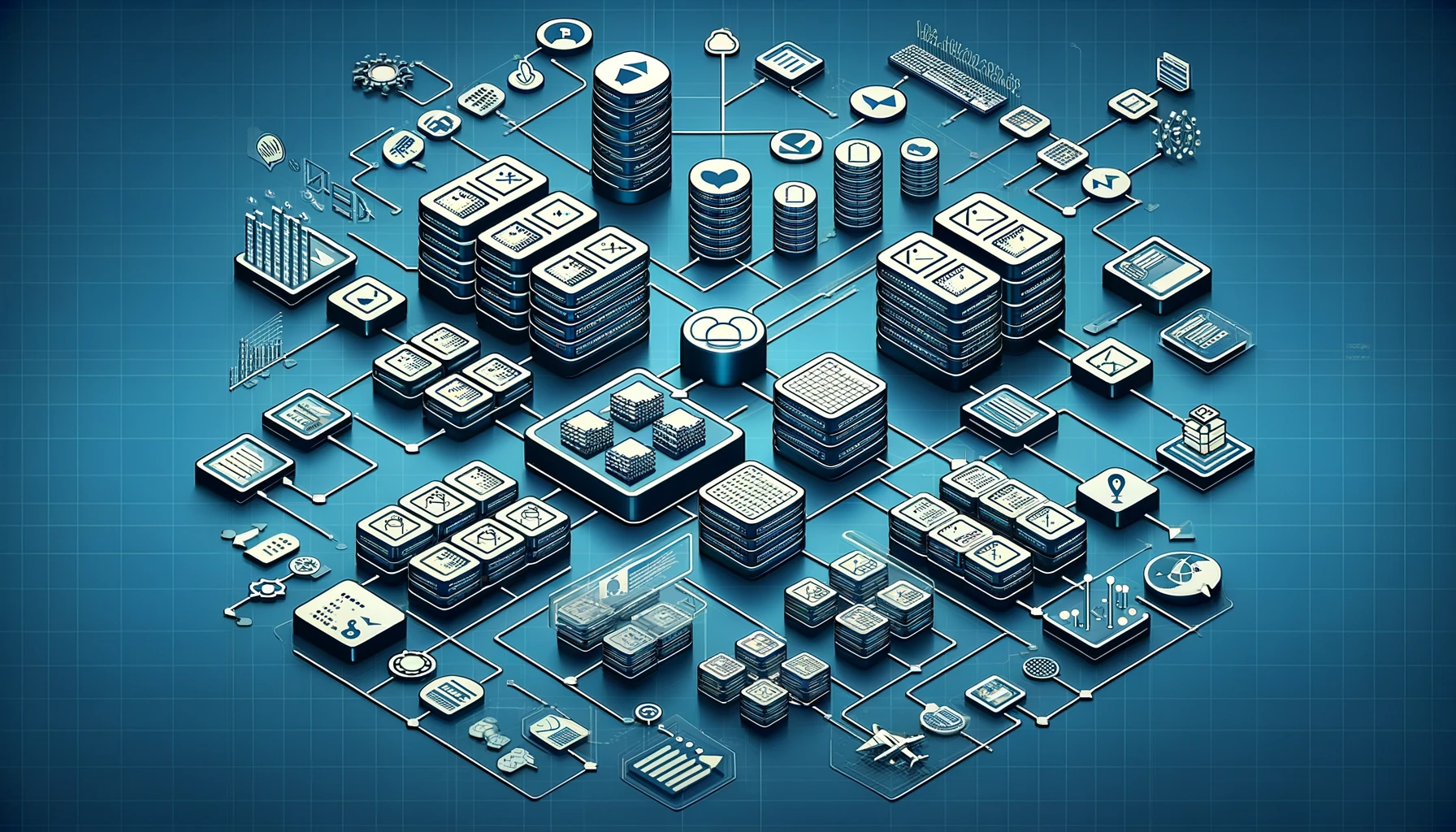